Programming #
This guide applies to version 2 of the EC-M11-BC-C1 module. The NORVI EC-M11-BC-C1 has a mini USB port for serial connection with the SoC for programming. Any ESP32-supported programming IDE can be used to program the controller. Follow this guide to programming NORVI ESP32-based controllers with the Arduino IDE.
SoC: ESP32-WROOM32
Programming Port: USB UART
Wiring Digital Inputs, Analog inputs, and RS485 #
8-pin and 3-pin connectors and wire harness #
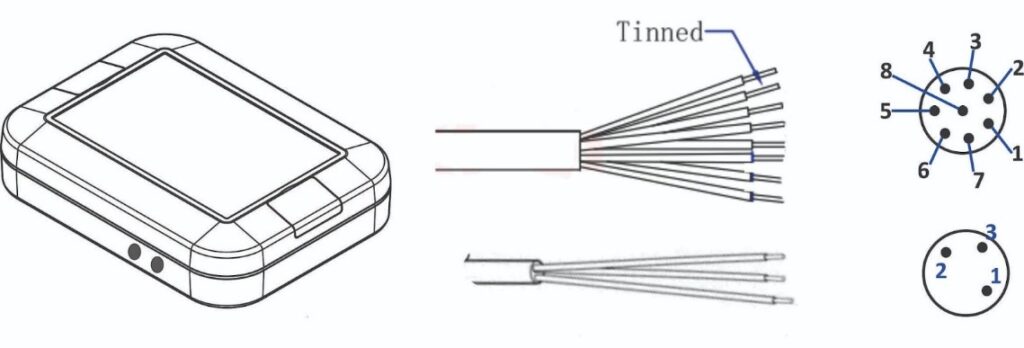
Pin Description #
8P Male | Wire color | I/O Configuration |
1 | White | Digital In A+ |
2 | Brown | Digital In A- |
3 | Green | Analog In 1 |
4 | Yellow | Analog In 2 |
5 | Gray | 12V+ |
6 | Pink | GND |
7 | Blue | – |
8 | Red | 3.3V |
3P Male | Wire color | I/O Configuration |
1 | Blue | Solar Panel + |
2 | Black | Not In Use |
3 | Brown | Solar Panel – |
Digital Inputs #
Wiring Digital Inputs #
The digital inputs of NORVI EC-M11-BC-C1 can be configured as both a sink and a source connection. The inverse of the digital input polar should be supplied to the common terminal.
Programming Digital Inputs #
Reading the relevant GPIO of the ESP32 gives the value of the digital input. When the inputs are in the OFF state, the GPIO goes HIGH, and when the inputs are in the ON state, the GPIO goes LOW. Refer to the GPIO allocation table in the datasheet for the digital input GPIO.
#define INPUT1 35
void setup() {
Serial.begin(115200);
Serial.println("Device Starting");
pinMode(INPUT1, INPUT);
}
void loop() {
Serial.print(digitalRead(INPUT1));
Serial.println("");
delay(500);
}
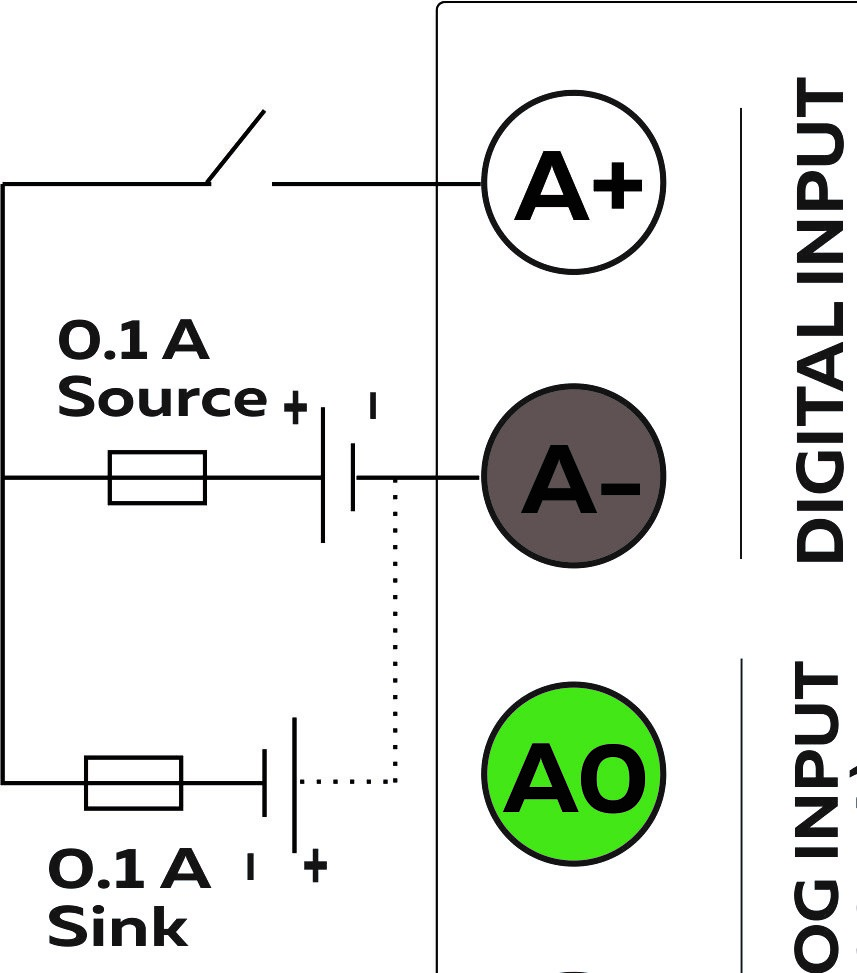
0-10 V Analog Input #
Reading Analog Input #
Analog Interrupt | GPIO21 |
Reading the relevant I2C address of the ADC gives the value of the analog input.
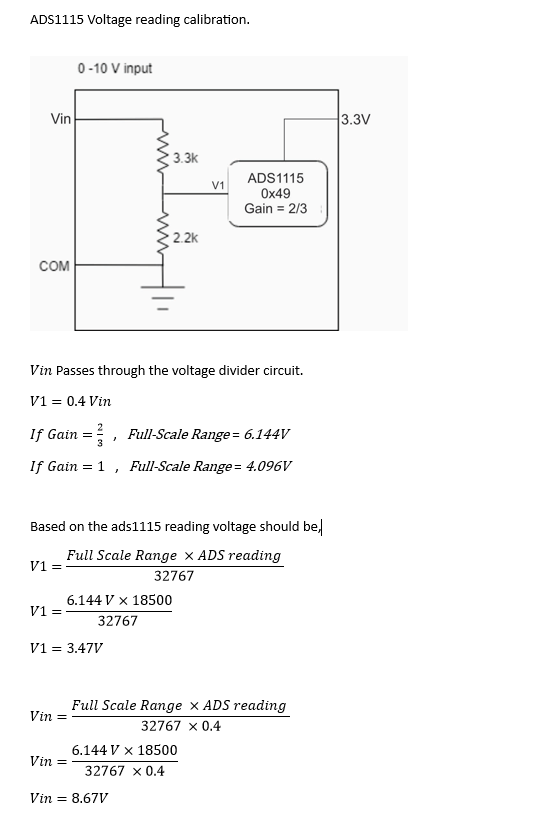
Programming Analog Inputs #
#include <Adafruit_ADS1015.h>
Adafruit_ADS1115 ads1(0x49);
String adcString[8];
void setup() {
Serial.begin(115200);
ads1.begin();
ads1.setGain(GAIN_ONE);
Serial.println("Initialized analog inputs");
}
void loop() {
Serial.print("Battery Voltage: ");
Serial.println(readBattery());
delay(800);
printanalog();
delay(800);
}
int readBattery() {
unsigned int analog_value;
analog_value = analogRead(36);
return analog_value;
}
void printanalog() {
adcString[0] = String(ads1.readADC_SingleEnded(0));
adcString[0] = String(ads1.readADC_SingleEnded(0));
delay(10);
adcString[1] = String(ads1.readADC_SingleEnded(1));
adcString[1] = String(ads1.readADC_SingleEnded(1));
delay(10);
adcString[2] = String(ads1.readADC_SingleEnded(2));
adcString[2] = String(ads1.readADC_SingleEnded(2));
delay(10);
adcString[3] = String(ads1.readADC_SingleEnded(3));
adcString[3] = String(ads1.readADC_SingleEnded(3));
delay(10);
Serial.print("A1: ");
Serial.print(adcString[0]);
Serial.print(" ");
Serial.print("A2: ");
Serial.println(adcString[1]);
Serial.print("A3: ");
Serial.print(adcString[2]);
Serial.print(" ");
Serial.print("A4: ");
Serial.println(adcString[3]);
Serial.println("____________________________________");
}
12V Power Output #
Enable | GPIO13 |
Programming power output #
#include <Adafruit_ADS1015.h>
Adafruit_ADS1115 ads1(0x49);
void setup() {
Serial.begin(115200);
pinMode(13, OUTPUT); // 12V boosted output enable
ads1.begin();
ads1.setGain(GAIN_ONE);
Serial.println("Initialized 12V battery power control");
}
void loop() {
digitalWrite(13, HIGH); // Enable 12V battery power
delay(800);
}
void disable12VBatteryPower() {
digitalWrite(13, LOW); // Disable 12V battery power
}
Solar Input #
Solar Powered Model | CN3083 |
Maximum Charge Current | 600mA |
Maximum Voltage | 6V |
Input Voltage monitor (V_SENSE2) | ADS1115 – 0x49 – AIN2 |
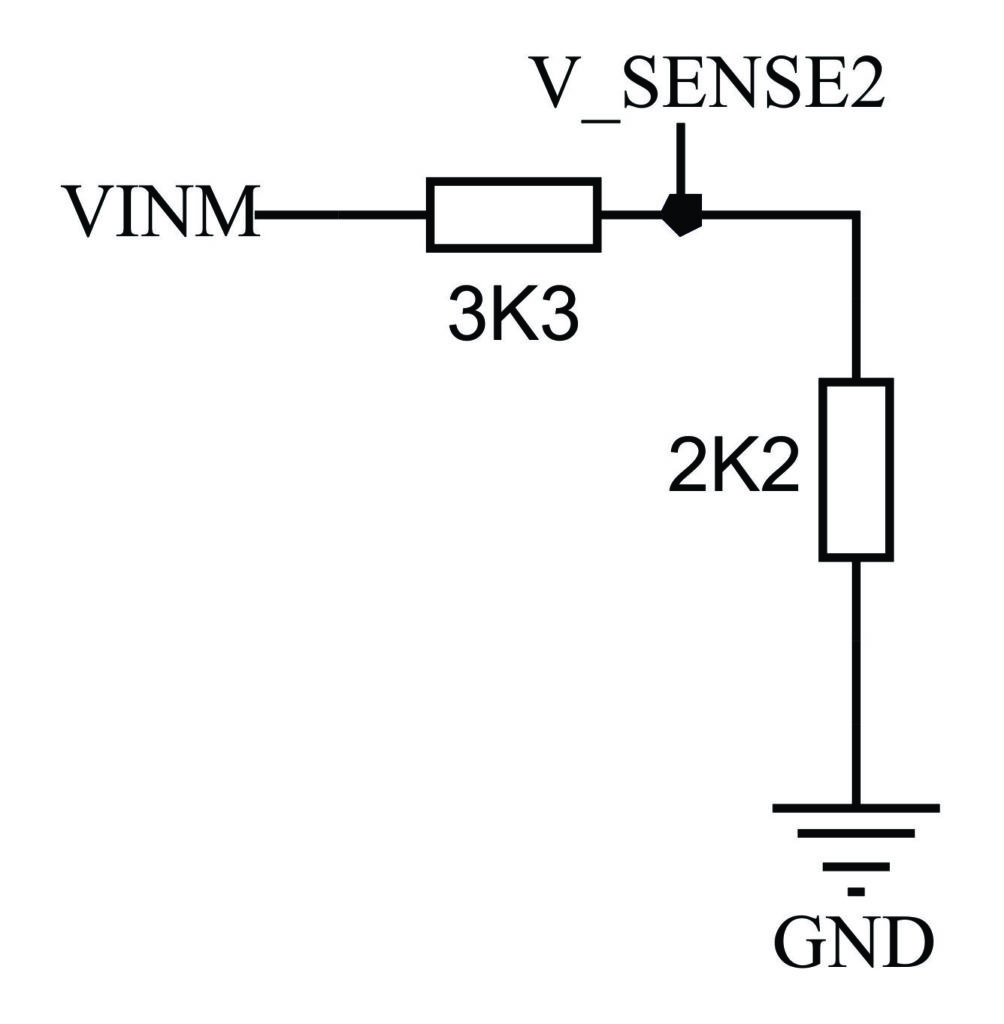
Battery Input #
Battery Type | 103040 Lithium polymer battery |
Nominal Capacity | 1200mAh |
Nominal Voltage | 3.75V |
Overcharge | 4.2V |
Over-discharge Cutoff Voltage | 3V |
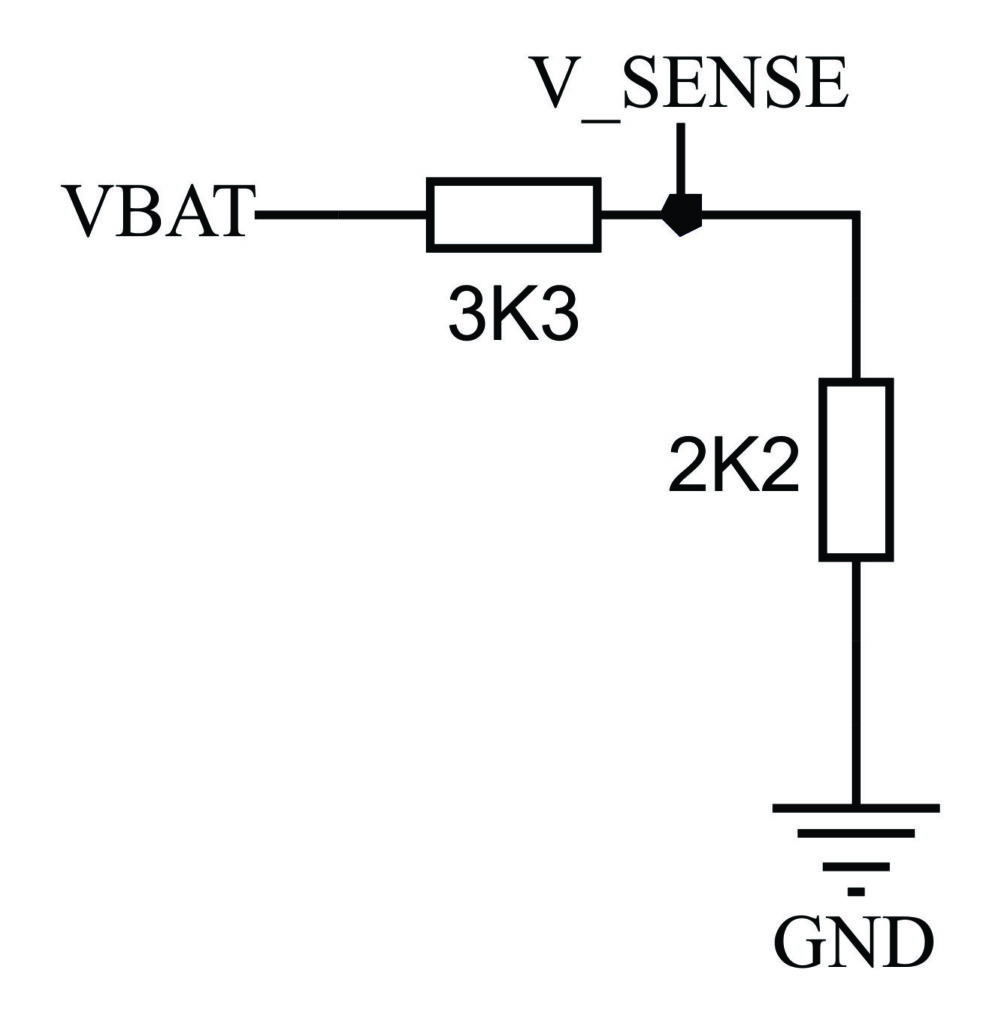
Programming Solar and Battery #
#include <Adafruit_SSD1306.h>
#include <Adafruit_ADS1X15.h>
Adafruit_ADS1115 ads1;
int analog_value = 0;
void setup() {
Serial.begin(115200);// put your setup code here, to run once:
Wire.begin(16,17);
if (!ads1.begin(0x49)) {
Serial.println("Failed to initialize ADS 1 .");
while (1);
}
}
void loop() {
int16_t adc0, adc1, adc2, adc3;
adc0 = ads1.readADC_SingleEnded(0);
adc1 = ads1.readADC_SingleEnded(1);
adc2 = ads1.readADC_SingleEnded(2);
adc3 = ads1.readADC_SingleEnded(3);
Serial.println("-----------------------------------------------------------");
Serial.print("AIN1: ");
Serial.print(adc0);
Serial.println(" ");
Serial.print("AIN2: ");
Serial.print(adc1);
Serial.println(" ");
Serial.print("SOLAR: ");
Serial.print(adc2);
Serial.println(" ");
Serial.print("AIN4: ");
Serial.print(adc3);
Serial.println(" ");
}